Documentation
Below you will find documentation on each of the components included in the Rebuy + Hydrogen package.
RebuyContextProvider
The RebuyContextProvider
is a React provider component. It is responsible for collecting environment and state-based information as a RebuyContext
. It will gather information about the current URL, session UTM parameters, as well as cart information such as the cart token, subtotal, line items, etc. As a provider component, no UI is rendered, it simply creates a React context value that can be requested by any child component.
The RebuyContextProvider
is a client component, which means it renders in the browser. It has three dependencies, which are useShop
, useUrl
, and useCart
, so the component should be located high up in the component tree but below the ShopifyProvider
and CartProvider
.
The recommended location is in src/App.server.jsx
inside <Router>
and wrapping both <FileRoutes>
and the <Route>
components, which makes the RebuyContext
automatically available on all pages across the site.
Import the RebuyContextProvider
import {RebuyContextProvider} from '@rebuy/rebuy-hydrogen/RebuyContextProvider.client';
Wrap the FileRoutes
and Route
components with RebuyContextProvider
FileRoutes
and Route
components with RebuyContextProvider
<Router>
<RebuyContextProvider>
<FileRoutes
basePath={countryCode ? `/${countryCode}/` : undefined}
routes={routes}
/>
<Route path="*" page={<NotFound />} />
</RebuyContextProvider>
</Router>
Access RebuyContext
Rebuy + Hydrogen components will automatically include and use RebuyContext
, but if you would like to use this context information in your own components, you certainly can!
import {RebuyContext} from '@rebuy/rebuy-hydrogen/RebuyContexts.client';
import {useContext} from 'react';
// Access context parameters with React's useContext hook
const {contextParameters} = useContext(RebuyContext);
RebuyWidgetContainer
The RebuyWidgetContainer
is a React container component. This component is responsible for fetching data and state management. It does not render any UI components, and will pass along its properties and data received from Rebuy to all of their children components. This abstraction allows you to use your existing application components or Rebuy's out of the box components.
Component properties
The component accepts arguments via props to allow for different API responses. All props listed below are optional, if no dataSource
prop is provided then '/api/v1/products/recommended'
will be used.
Property | Value |
---|---|
dataSource | {String} A relative URL path of your Rebuy data source (i.e. /api/v1/products/recommended ) |
product | {Object} A product object with an id |
productId | {String} A product ID string in GraphQL URL format |
variant | {Object} A variant object with an id |
variantId | {String} A variant ID string in GraphQL URL format |
collection | {Object} A collection object with an id |
collectionId | {String} A collection ID string in GraphQL URL format |
limit | {Number} A number of products to return from Rebuy's API |
options | {Object} An object with key, value pairs of Rebuy REST API arguments (i.e. metafields: "yes" ) |
Children components properties
All child components will inherit the container props, in addition to two container generated props products
and metadata
. So if you've provided a product={product}
prop to the container, then each child component will have the following properties product
, products
, and metadata
.
Property | Value |
---|---|
products | {Array} An array of product objects returned from Rebuy's API |
metadata | {Object} An object with metadata about the input data, rules matched, rules not matched, excluded products, and cache info |
Example implementation
In the following example, we are using the RebuyProductRecommendations
component which is a UI component used to render data provided to it via props by RebuyWidgetContainer
.
When the components are rendered, it will display 6
products powered by Rebuy's AI algorithm based on the provided input product
. So if the input product was ketchup, you would likely see recommended products like mustard, mayo, or other condiments.
import {RebuyProductRecommendations} from '@rebuy/rebuy-hydrogen/RebuyProductRecommendations.client';
import {RebuyWidgetContainer} from '@rebuy/rebuy-hydrogen/RebuyWidgetContainer.client';
<ProductOptionsProvider data={product}>
{/* ... */}
{/* REBUY: Product Recommendations */}
<Suspense>
<RebuyWidgetContainer
product={product}
dataSource="/api/v1/products/recommended"
limit="6"
>
<RebuyProductRecommendations title="My custom title" />
</RebuyWidgetContainer>
</Suspense>
</ProductOptionsProvider>
RebuyProductViewed
RebuyProductViewed
is a client component, which helps automate customer browsing behavior. This behavior is used to train the AI as well as power recently viewed products. This component would typically live in your products route file located at ./src/routes/products/[handle].server.jsx
, so as a customer is browsing your site we automatically record the product pages they are viewing.
Component properties
The component accepts arguments via props to allow for registering different products that have been viewed. One of the following properties are required to fire the event:
Property | Value |
---|---|
product | {Object} A product object with an id |
productId | {String} A product ID string in GraphQL URL format |
productHandle | {String} A product handle string |
Example implementation
The following example would be included in the products route file at products/[handle].server.jsx
to automatically register customer product view events. The current product displayed is passed to the RebuyProductViewed
component via props as product={product}
.
import {RebuyProductViewed} from '@rebuy/rebuy-hydrogen/RebuyProductViewed.client';
<ProductOptionsProvider data={product}>
{/* ... */}
<Suspense>
<RebuyProductViewed product={product} />
</Suspense>
</ProductOptionsProvider>
RebuyProductRecommendations
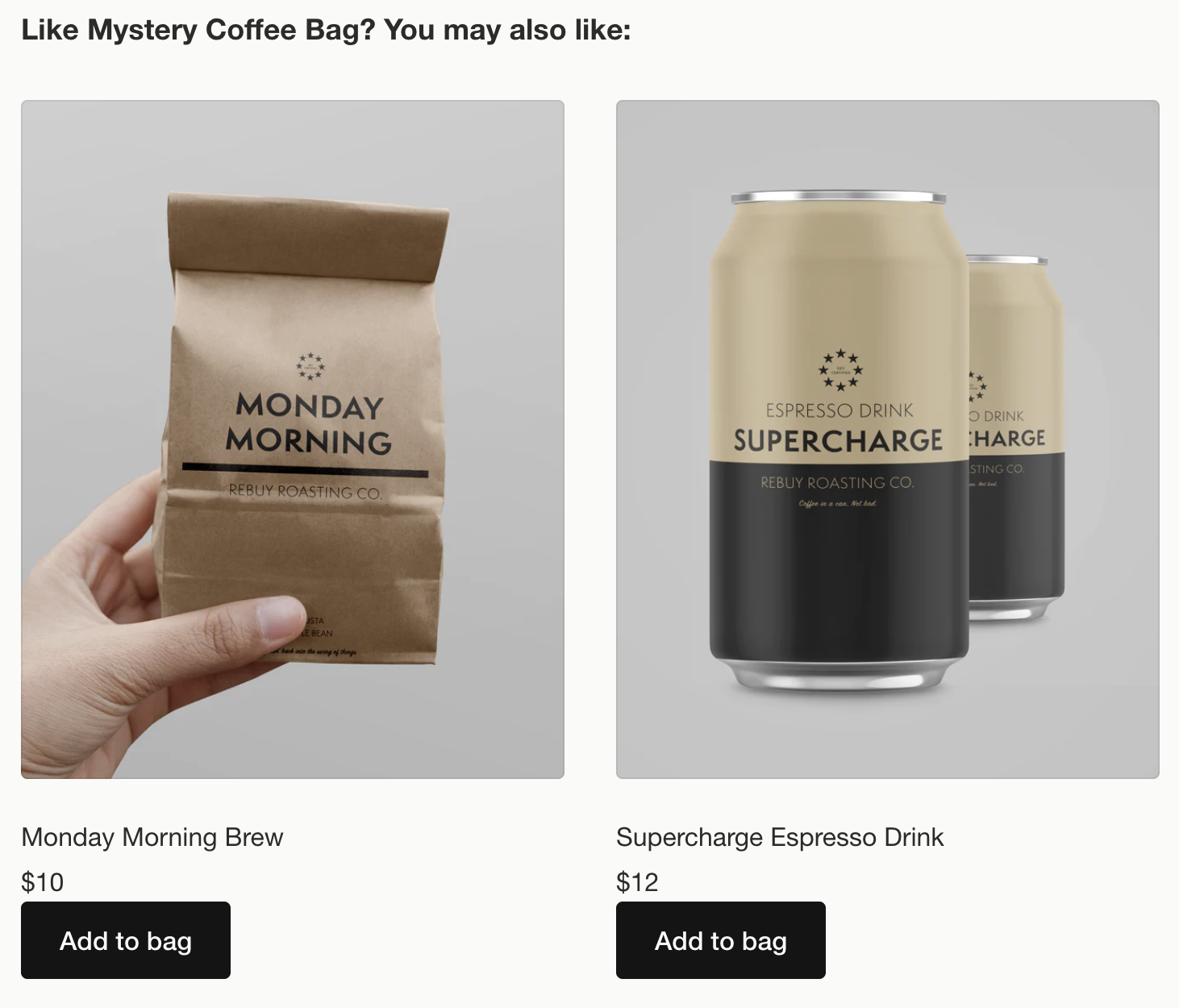
Recommending similar products personalized for you store
RebuyProductRecommendations
is a UI component used to render AI-driven product recommendations provided to it via props by the RebuyWidgetContainer
component.
Component properties
See RebuyWidgetContainer
children components properties above, including these additional props:
Property | Value |
---|---|
title | {String} An optional component title |
Example implementation
See RebuyWidgetContainer
example implementation above.
RebuyRecentlyViewedProducts
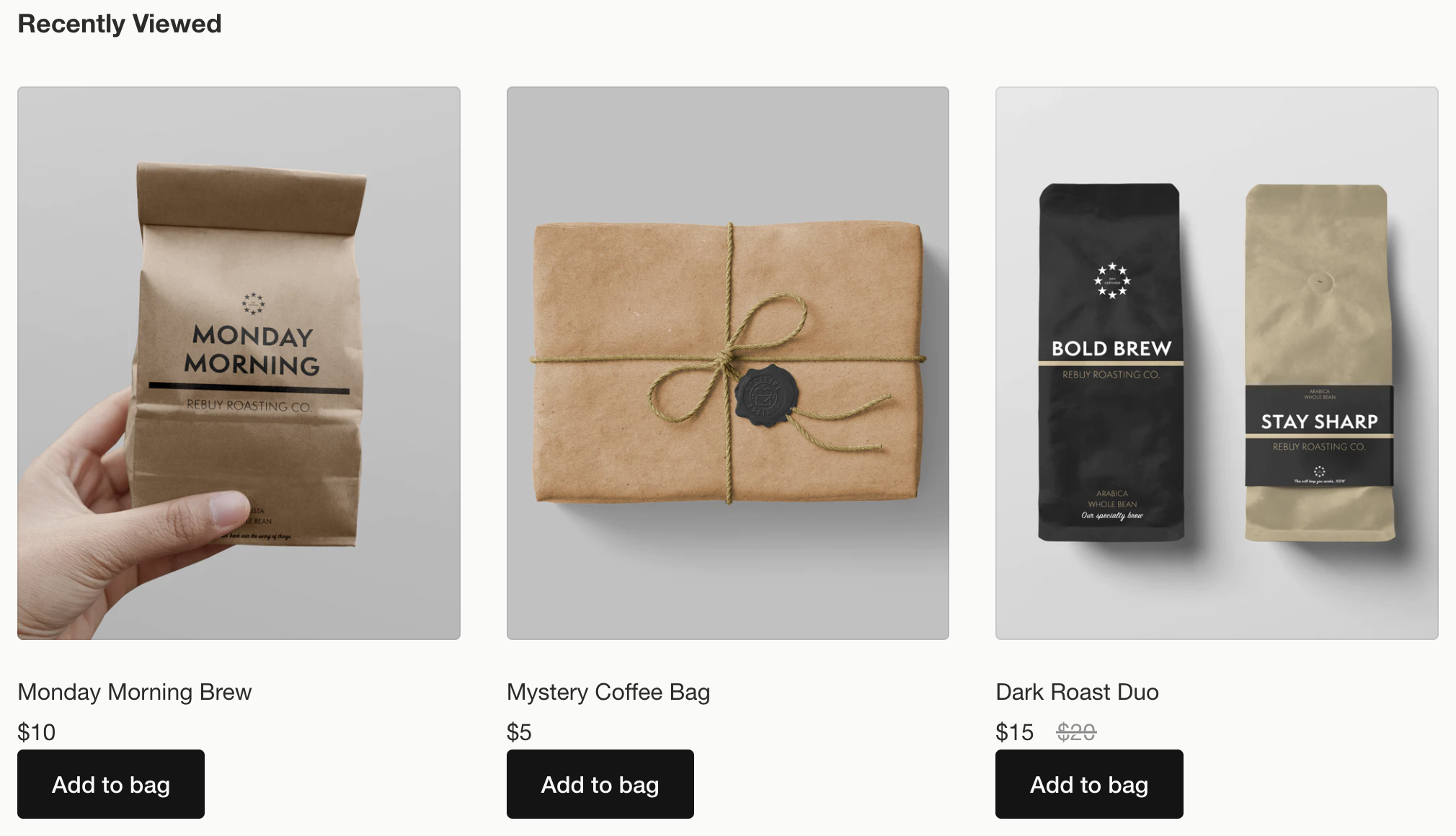
Automatically populated with most recently viewed products with option to quickly add to cart
RebuyRecentlyViewedProducts
is a UI component used to render products that a visitor has viewed recently while navigating your site. The necessary event tracking is automatically managed by Rebuy provided you have also implemented the RebuyProductViewed
component on your products pages.
Component properties
See RebuyWidgetContainer
children components properties above.
Example implementation
See RebuyWidgetContainer
example implementation above.
RebuyCompleteTheLook
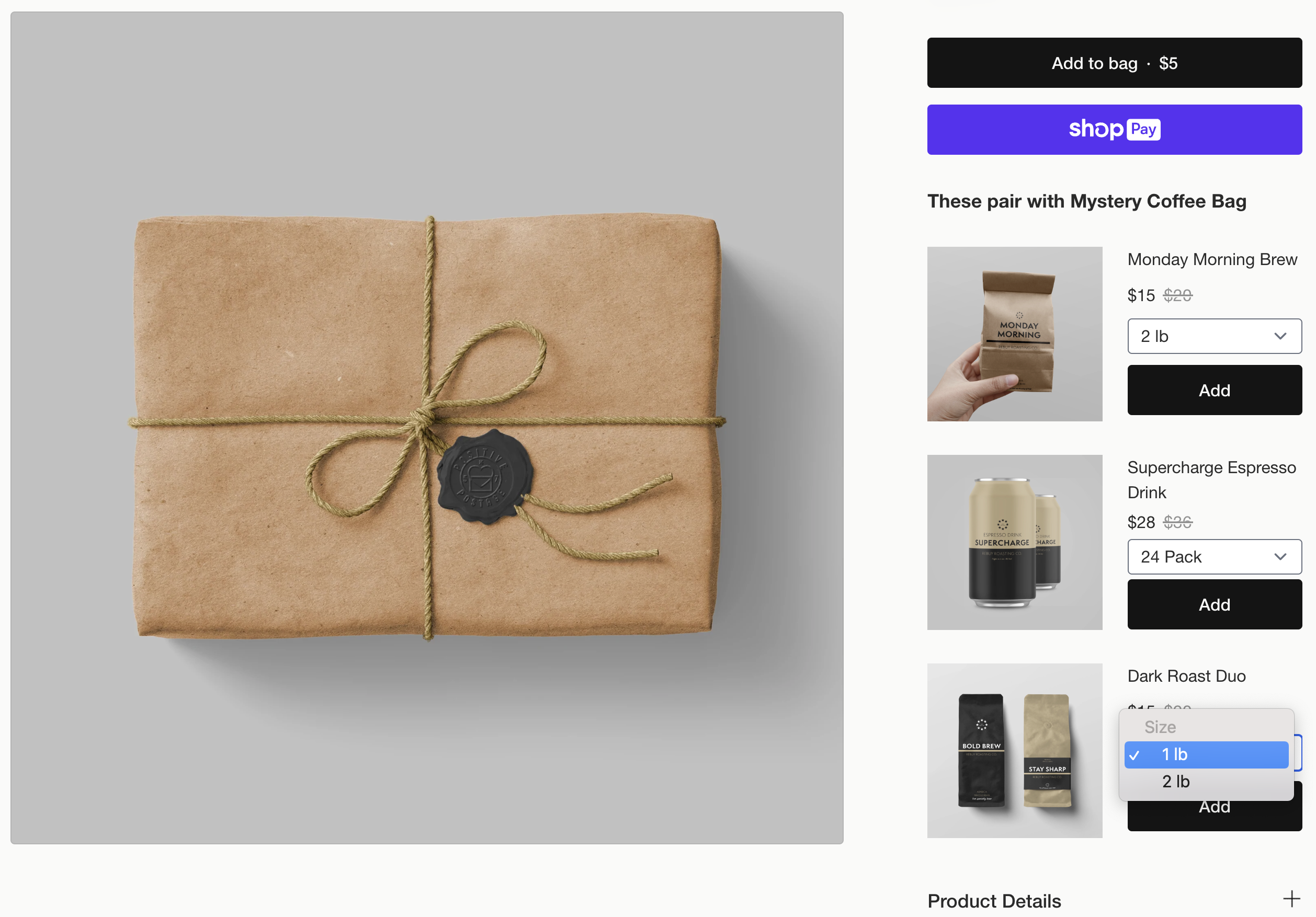
Recommend complimentary products that can be individually added to the cart
RebuyCompleteTheLook
is a UI component designed to live alongside the primary ATC button on your product pages, rendering product recommendations powered by Rebuy's AI and intended to show products that pair well together.
For example, if the input product was jeans, you might see recommended products like t-shirt, leather belt, or similar items that would compliment the current product and help to "complete the look."
Component properties
See RebuyWidgetContainer
children components properties above, including these additional props:
Property | Value |
---|---|
title | {String} An optional component title |
className | {String} An optional list of CSS classes to include on the component |
Example implementation
See RebuyWidgetContainer
example implementation above.
RebuyProductAddOns
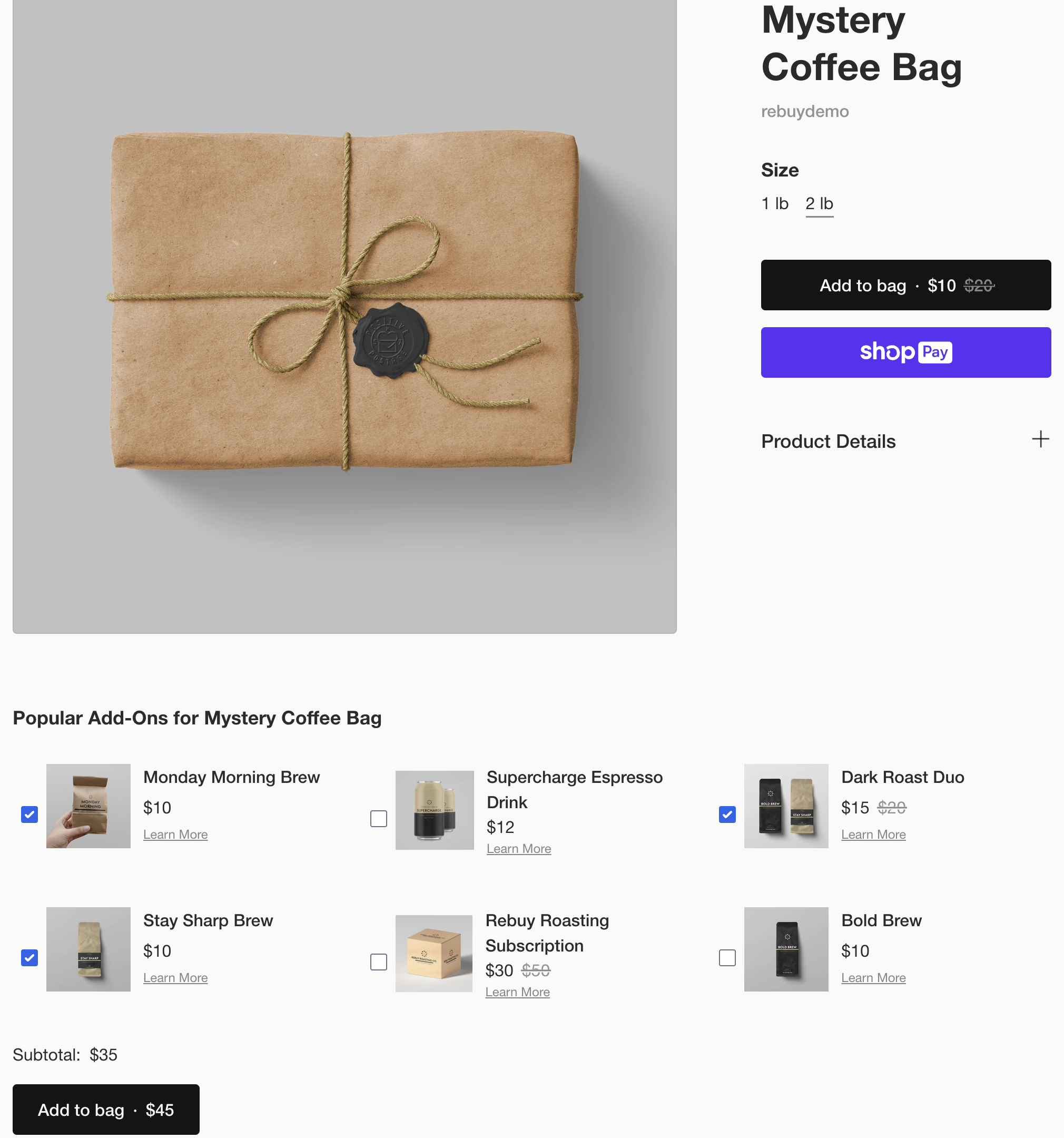
Choose which add-on products also get added to the cart allowing for custom bundling
RebuyProductAddOns
is a UI component used to render AI-driven product recommendations than can be added to the cart together, including the current product being displayed. Customers can optionally select which add-on products they would like to be included, and a subtotal for each selected add-on is displayed alongside the total cost before they "Add to cart."
Component properties
See RebuyWidgetContainer
children components properties above, including these additional props:
Property | Value |
---|---|
title | {String} An optional component title |
className | {String} An optional list of CSS classes to include on the component |
Example implementation
See RebuyWidgetContainer
example implementation above.
RebuyDynamicBundleProducts
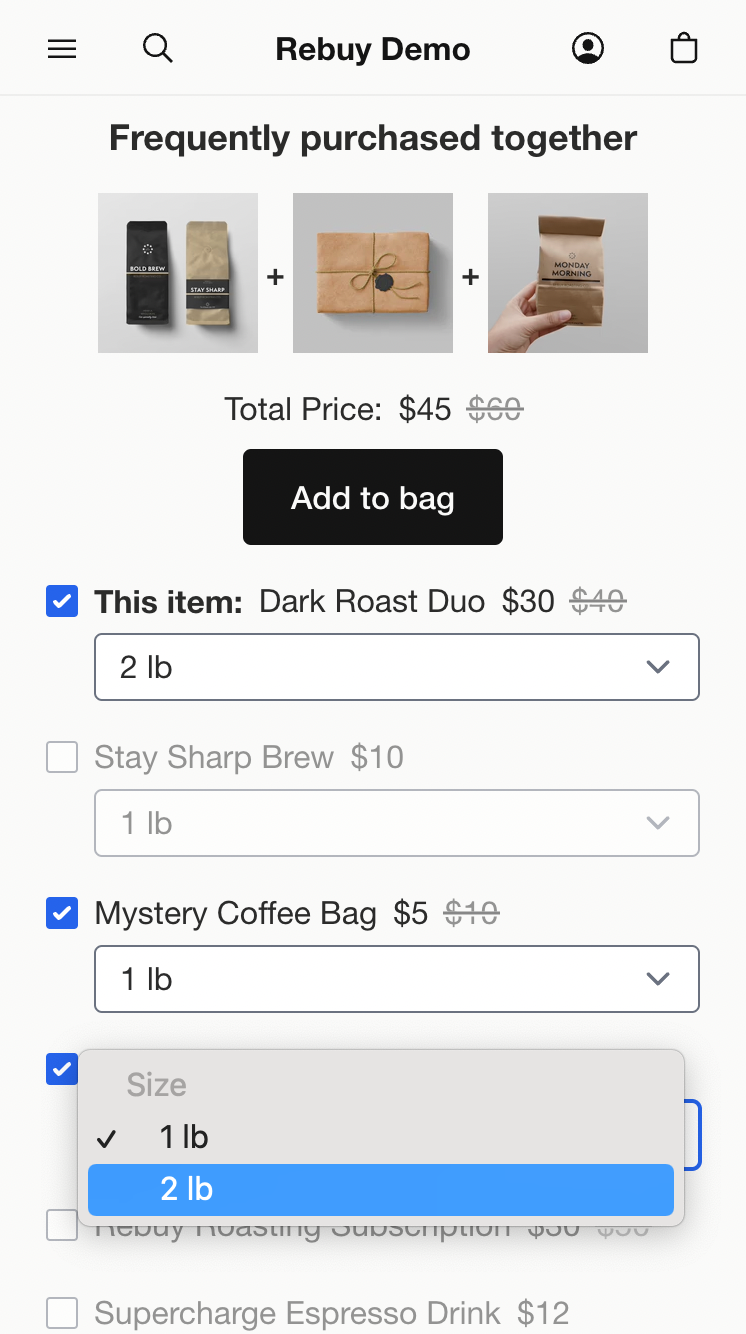
Personalized dynamic bundle featuring product variant selection
RebuyDynamicBundleProducts
is a UI component used to render complimentary recommendations driven by Rebuy's AI that can be bundled together. Each recommended product, including the product being viewed, can selectively be included or removed from the dynamic bundle, allowing the bundled items to be added to the cart in a single motion. This component also supports variant selection, and any out of stock product variants are accounted for when preparing the dynamic bundle.
When customers select which products they would like to be part of their personalized bundle, the total cost (including any cumulative compareAt discounts) for their selected configuration is displayed above the "Add to cart" button.
Component properties
See RebuyWidgetContainer
children components properties above, including these additional props:
Property | Value |
---|---|
title | {String} An optional component title |
className | {String} An optional list of CSS classes to include on the component |
Example implementation
See RebuyWidgetContainer
example implementation above.